# Exploring Developer Mode on the Xbox Series X
I've been following Modern Vintage Gamer on youtube for a while and saw this video https://www.youtube.com/watch?v=UGAjhjp8dnI
For $19 you can enable Developer Mode on an Xbox Series S/X and try to make your own games.
In MVG's video he used this to deploy a version of RetroArch that's able to emulate a ton of games. So I went down this path.
It's not all sunshine and roses. There were a few things he didn't mention and to be honest it's kind of janky. Things like to crash or not work at all.
I liked this video a lot https://www.youtube.com/watch?v=mj9MPuywjmo as he explained a bunch of stuff MVG left out.
To save you some time here are some things I found:
- After running the Developer mode app. It complained about needing an update. Using this secret shortcut got it to continue. -> https://stackoverflow.com/questions/63825400/how-to-enable-xbox-one-developer-mode-activation
- Once in developer mode you need to enable remote access and disable authentication (bottom right of the main screen)
- Pressing start on the main screen allows you to manage storage and give more than 5gB to developer mode
- The Xbox Device Portal url http://ip:11443 was designed for Internet Explorer 11. Uploading files from my mac tended to not work.
- Uploading files does not provide a progress bar
- The browse button on the Xbox Device Portal gives you instructions on how to use SMB to transfer files
- Once you launch RetroArch you need to update from the menus to work properly and then close and restart it
- UWP apps can only see files in certain directories that belong to that App and they have some stupid long names
- Files on USB's can't be >2gB
- When you deploy apps if you press Back under view details, you can change them from App to Game which gives them more system resources
After playing with this for a bit, this got me thinking, what else can I do in developer mode.
You can compile your own UWP Apps and run them https://github.com/microsoft/Windows-universal-samples Keep in mind UWP does not support a lot of win32 functions.
I found you can install a variety of UWP apps even if they weren't meant to be on an xbox using https://store.rg-adguard.net/ and downloading the appxbundle or msixbundle.
Not every app works. You just gotta try them out. Here are some working ones.
- Remote Desktop Client: https://www.microsoft.com/en-us/p/microsoft-remote-desktop/9wzdncrfj3ps
- HTTP Server: https://www.microsoft.com/en-us/p/http-server-host-static-webpages/9nkjdc855r3f
- FTP Server: https://www.microsoft.com/en-us/p/universal-ftp-server/9nqkq104hb9r
I was able able to install fluent-terminal, but didn't have any luck getting a terminal to display. https://github.com/felixse/FluentTerminal
They have crypto currency miners, but I didn't have any luck. Honestly I'm kind of surprised I haven't heard more of people using this to do crypto currency mining.
- Bitcoin Miner: https://www.microsoft.com/en-us/p/app/9wzdncrdhxb6 (Needs appx dependency as well) (Runs but does not connect to pool properly)
- Ethereum Miner: https://www.microsoft.com/en-us/p/mine-eth/9nrsfdw8h837 (Crashes on loading)
The visual studio pin is actually an SSH password for the `DevToolsUser`.
```
ssh DevToolsUser@ip
```
There was an exploit released in June 2019 for the Xbox Dev mode to get a system account, but it has since been patched. https://www.realmodscene.com/index.php?/topic/8907-xbox-one-dev-mode-shell-and-win32-code-execution/
With some research I found:
https://webcache.googleusercontent.com/search?q=cache:5amqbLKgHhkJ:https://billyhulbert.github.io/page3/+&cd=1&hl=en&ct=clnk&gl=us
https://github.com/billyhulbert/XboxUnattend
The superfun writeup shows a few cool things you can do and provides some background.
I'm not sure how superfun.exe was compiled, but it executes. The XRF template provided does not execute when compiled.
I learned in this mode the xbox is running three different operating systems (game, system, host) and has a very limited shell without privileges to do much.
Once you connect there are a handful drives you can access by drive letter.
```
C: D: E: J: M: N: O: Q: S: T: U: V: X: Y:
```
```
C:\ -> System.xvd
D:\ -> Development scratch for dev-mode
E:\ -> USB
J:\ -> SystemTools.xvd (dev-mode only)
M:\ -> SystemMisc.xvd
N:\ -> idk?
O:\ -> Bluray Drive
Q:\ -> Users folders
S:\ -> Settings.xvd | Settings-devkit.xvd
T:\ -> Temp.xvd
U:\ -> user.xvd / user-devkit.xvd
X:\ -> SystemAux.xvd
Y:\ -> SystemAuxF.xvd
```
Binaries for your apps you deploy live in:
```
D:\DevelopmentFiles\WindowsApps>
```
You can write to
```
D:\Temp
```
From SMB this is:
```
\\IP\SystemScratch\Temp\
```
The E drive is the USB you connected. Although you can't write to it.
Under `C:\windows\system32\` you can see a set of binaries
You can launch a telnetd service on port 24 using:
```
devtoolslauncher LaunchForProfiling telnetd "cmd.exe 24"
```
You can run python by extracting the embedded version from https://www.python.org/ftp/python/3.9.1/python-3.9.1-embed-amd64.zip
Uncomment `import site` in `python39._pth`

You can install pip from get-pip
```
set PATH="%PATH%;D:\Temp\python-3.9.1-embed-amd64"
set PATH="%PATH%;D:\Temp\python-3.9.1-embed-amd64\scripts"
set PTYHONPATH="D:\Temp\python-3.9.1-embed-amd64"
python get-pip.py
```
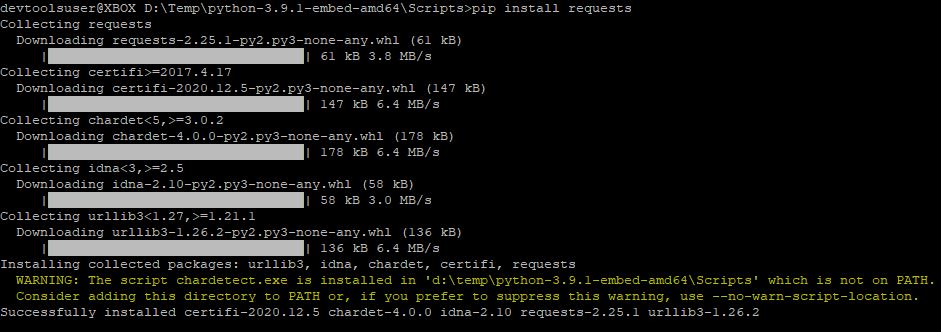
You can compile golang binaries and run them make sure to compile as x64
```
package main
import "fmt"
func main() {
fmt.Println("hello world")
}
go build main.go
```

Powershell works as well from https://github.com/PowerShell/PowerShell/releases/download/v7.1.1/PowerShell-7.1.1-win-x64.zip
From what I've seen calling Win32 API's from wmic and gcim do not work.
C# and C++ Hello worlds compiled in visual studio will not work, but gcc does
```
#include
#include
void main()
{
printf("Hello\n");
MEMORYSTATUSEX memInfo;
memInfo.dwLength = sizeof(MEMORYSTATUSEX);
GlobalMemoryStatusEx(&memInfo);
int mem = memInfo.ullTotalPhys / (1024*1024);
printf("Memory: %d",mem);
}
```
```
x86_64-w64-mingw32-gcc memory.c -o memory.exe
```
Apps run from SSH appear to only have access to 1536mB of ram. Apps in game mode have 5gB.
I found you can statically compile dotnetcore applications using il compiler -> https://www.codeproject.com/Articles/5262251/Generate-Native-Executable-from-NET-Core-3-1-Proje
I haven't found a way to run dotnet framework applications yet or C/C++ made in visual studio.
Running https://github.com/consen/demo/blob/master/c/syntax/asm/cpuid.c I get
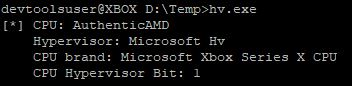
Running https://github.com/klauspost/cpuid I get

Powershell `get-process` lists running processes
Attempting to create a vnc service and connecting back to my server shows a connection, but it resets. Probably because there's no desktop to connect to.

Running the new kernelconnect bug crashes my xbox https://www.youtube.com/watch?v=JjebNlzX6us
```
\\.\globalroot\device\condrv\kernelconnect
```
I figured out how to compile c/c++ in visual studio to make binaries that execute. So you need to include the correct libraries.
https://github.com/billyhulbert/XboxUnattend
```
mkdir Build
cd Build
cmake ..\ -G "Visual Studio 16 2019"
cmake --build . --config Release
```
You can modify the code after the libraries are setup properly.
I think that's enough fun for today.
If you care more about xbox hacking it would be worth looking at some of these repos: https://github.com/tunip3?tab=repositories